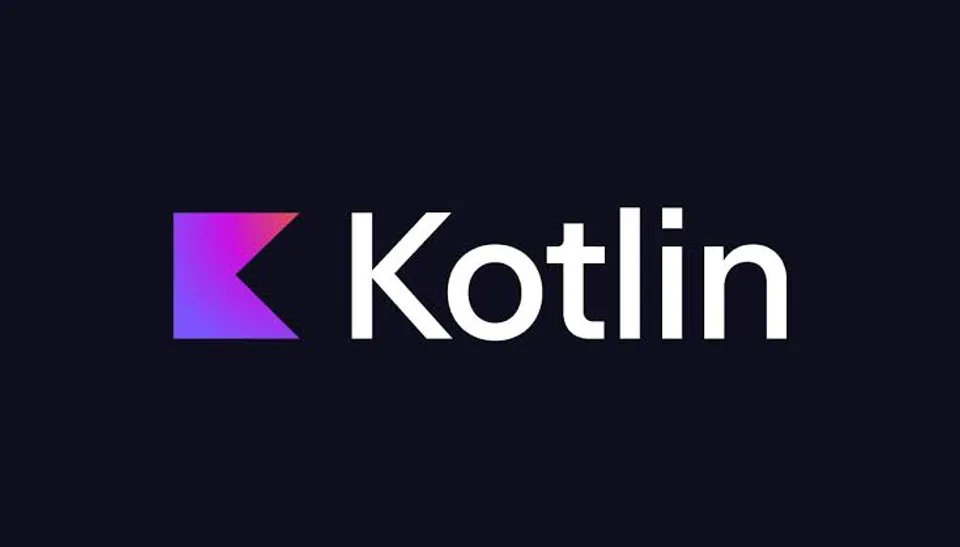
Part 2: Kotlin Fundamentals: Foundation for Beginners
Part 2 : Basic Things You Need to Know! 🚀
Kali ini gue bakal deep dive ke Kotlin nih. FYI, Kotlin tuh bahasa programming yang lagi hot banget, especially buat bikin aplikasi Android. Trust me, once you get it, bakal auto suka! 💯
Yang Bakal Lo Pelajarin
- Basic Stuff: Variables & Data Types
- Control Flow: Biar Code Lo Gak Monoton
- Functions: Bikin Code Lo More Organized
- Null Safety: Biar App Lo Gak Crash
- Collections: Data Structure but Make It Simple
- Next Level Stuff
Basic Stuff: Variables & Data Types 📱
Variables be Like
Di Kotlin ada 2 tipe variable ya guys:
val
-> ini tuh kyk crush lo yang impossible berubah (read-only)var
-> nah kalo ini kyk mood lo, bisa berubah-ubah
// Crush lo (gak bisa diubah)
val crush = "Jung Kook"
// Mood lo (bisa diubah)
var mood = "Happy"
mood = "Sad" // masih valid kok
Data Types yang Sering Dipake
Tipe | Contoh | IRL Example |
---|---|---|
Int | val followers: Int = 1000 | Jumlah followers lo |
Double | val rating: Double = 4.5 | Rating resto |
String | val bio: String = "Living my best life" | Caption Instagram |
Boolean | val isNgoding: Boolean = true | Status WA lo pas lagi ngoding |
Control Flow: Make It Flow! 🌊
If-Else but Make It Kotlin
val yourFollowers = 999
val status = if (yourFollowers > 1000) {
"Lo udah jadi micro influencer!"
} else {
"Keep grinding bestie!"
}
When Expression (Switch Case’s Cool Cousin)
val day = 3
val schedule = when (day) {
1 -> "Senin: Ngampus 😫"
2 -> "Selasa: Ngoding 💻"
3 -> "Rabu: Touch some grass 🌱"
4 -> "Kamis: Gym reveal 💪"
5 -> "Jumat: Finally weekend! 🎉"
else -> "Weekend: Netflix & ngoding"
}
Functions: Your Code’s Best Friend 🤝
// Basic function
fun sayHi(name: String = "bestie") {
println("Hai $name, welcome to the coding zone!")
}
// Function with output
fun hitungDiskon(harga: Int, diskon: Int = 10): Int {
return harga - (harga * diskon / 100)
}
Null Safety: No More App Crash! 🛡️
// Bikin variable yang bisa null
var crush: String? = null
// Safe call biar gak anxiety
println(crush?.length) // Aman, gak bakal crash
// Elvis operator (?:) - kasih default value
val crushNameLength = crush?.length ?: 0 // Kalo null, pake 0
Collections: Data Structure but Simplified 📚
List (Kyk Playlist Spotify Lo)
// List yang gak bisa diubah
val playlist = listOf("Taylor Swift", "NewJeans", "BLACKPINK")
// List yang bisa diubah
val dailyMood = mutableListOf("Happy", "Blessed", "Sleepy")
dailyMood.add("Hungry") // Totally valid
Map (Key-Value Things)
val social = mapOf(
"ig" to "@koding.asik",
"followers" to 10000,
"isVerified" to false
)
Next Level Stuff 🚀
Higher-Order Functions
// Function yang bisa terima function lain as parameter
fun doMoodCheck(mood: String, action: (String) -> String) {
val result = action(mood)
println("Mood lo: $result")
}
// Pakenya gini
doMoodCheck("happy") { mood ->
"Feeling $mood, might delete later ✨"
}
Tips Buat Lo Yang Mau Jago Kotlin! 💡
-
Start Small
- Mulai dari basic dulu
- Build small projects
- Share progress lo di social media
-
Practice Makes Perfect
- Ngoding tiap hari (minimal 1 jam)
- Ikut coding challenge
- Join komunitas Kotlin
-
Resources Yang Worth It
- Kotlin Official Website
- Kotlin Playground
- Join Discord/Telegram komunitas Kotlin Indonesia
Wrap Up 🎯
Nah itu dia basic Kotlin yang perlu lo tau! Remember ya guys:
- Take your time to learn
- It’s okay to make mistakes
- Stack Overflow is your friend
- Don’t forget to touch grass
Keep grinding! Semoga tutorial ini helpful buat lo yang mau jadi developer Android kece. Drop comment kalo ada yang mau ditanyain!
“Ngoding itu kyk relationship, kadang bikin stress tapi worth it in the end” - Developer’s Life 💻✨